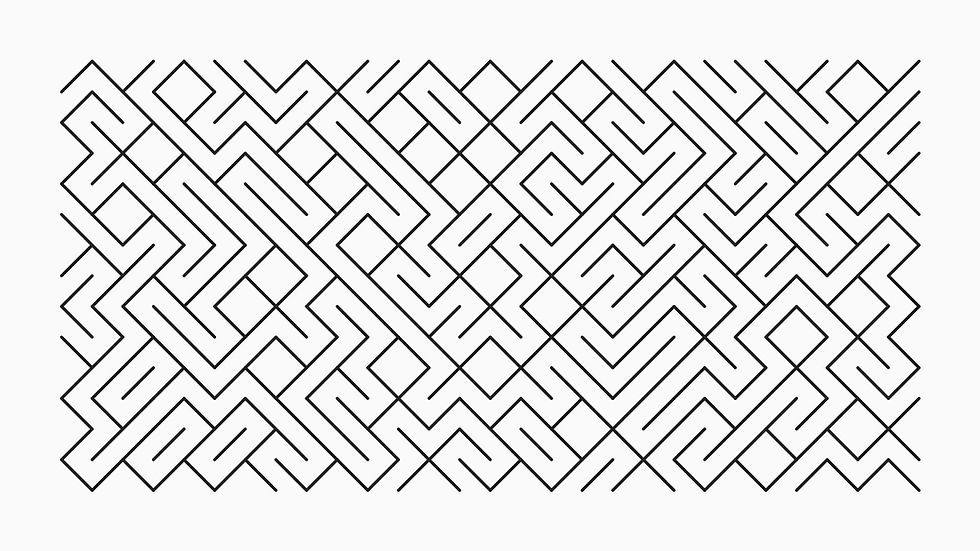
What you will learn
In this tutorial, we'll focus on creating abstract mazes, a mesmerizing form of generative art. With each run, your code will birth a new maze like abstract art, each one unique and captivating. Throughout the tutorial, you will gain proficiency in the following key concepts:
Canvas Tiling: Learn how to divide your canvas into tiles, laying the foundation for the intricate patterns that will compose your maze.
Random Number Generation: Dive into the world of randomness as we generate numbers to introduce variability, ensuring that no two mazes are alike.
Statistics in Art: Uncover the artistic potential of statistical analysis as we manipulate random values to craft balanced and visually appealing mazes.
Basic p5.js Commands: Master fundamental p5.js commands to draw shapes, lines, and patterns, providing you with the tools to bring your abstract maze to life.
General idea of the artwork
I will divide the canvas into cells. in each cell the computer will generate a diagonal line that either slants upwards or downwards.
If the diagonal slants upwards, the start is at the top left corner of the cell and the end at the bottom right corner. If we will look at it as a cell with size 1x1 - the start at (0,0) and end at (1,1).
If the diagonal slants downwards the start is at the top right corner of the cell (1,0) and the end at the bottom left corner (0,1).
you can see that the direction impacts the first digit of the start and end location in an inverse relation.
Setting your environment
I. Installing and Configuring p5.js
Download Processing (Optional):
If you prefer working locally, visit www.processing.org to download Processing.
Follow the installation instructions for your operating system.
Online Editor (Recommended):
An accessible alternative is the online editor at https://editor.p5js.org/.
Open your web browser and navigate to the provided link.
II. Setting Up the Canvas
Initialize the Canvas:
Open a new p5.js sketch in your chosen environment.
Set up the width and height of your canvas, for the example I used the createCanvas ( windowWidth , windowHeight ); function to set it to my window size. replace windowWidth with the desired width and windowHeight with the desired height in pixels.
Background and Stroke:
Choose a background color with background ( color( "#FAFAFA" ) );
Configure the stroke weight using strokeWeight(5);
Set the stroke color with stroke ( color( "#202020" ) );
your code should look like this
function setup() {
createCanvas (windowWidth , windowHeight );
background ( color ("#FAFAFA" ) ) ;
strokeWeight( 5 );
stroke ( color( "#202020" ) );
}
III. Setting constants
I will be dividing the canvas into tiles lets set d as the tile size, and pad as the gap from the edge of canvas
function setup() {
createCanvas (windowWidth , windowHeight );
background ( color ("#FAFAFA" ) ) ;
strokeWeight( 5 );
stroke ( color( "#202020" ) );
}
const d = 50;
const pad = 1;
IV. dividing the canvas to cells
using for loops I will "run" across the width and height of the canvas. each step will be in the size of "d" (width of the cell). the stop condition for the loop will be when it will reach the width subtracted with "pad".
In order to verify that the cells are set properly i will draw a dot on each cell corner with point(x,y) function.
function draw() {
for (let i = pad; i*d <width - pad*d; i++) {
for (let j = pad; j * d < height - pad*d ; j++) {
point ( i*d , j*d );
}
}
noLoop();
V. Generate random lines
Initially, I will generate a random number, either "0" or "1". This number will determine the alternation of the line's start and end coordinates.
I will generate a random integer between 0 and 9999. Subsequently, I will calculate the modulo of 2 (the remainder when dividing by 2) for this number. The outcome, either "0" or "1," will be stored in the variable "r."
Using the value of "r," I will determine the start and end points as follows:
If the modulo is 0: Start at (0, 0), end at (1, 1).
If the modulo is 1: Start at (1, 0), end at (0, 1).
Therefore, the mathematical expression for the x-location will be:
For the start location: x0+r
For the end location: x0+1−r
I will substitute x0 with the iteration over the x-axis, and then multiply the result by "d" to represent the cell width.
the resulting code:
function draw() {
for (let i = pad; i*d <width - pad*d; i++) {
for (let j = pad; j * d < height - pad*d ; j++) {
let r = floor(random(9999)%2);
line(i*d +r*d, j*d, (i+1)*d -r*d , (j+1)*d);
}
}
noLoop();
}
now run your code and enjoy the results.
you can customize the parameters such as cell width , stroke width, colors, etc. to create your own unique generative art.
I would love to see your art, join my forums and share share your artwork.
Aaron!
Comments